Introduction to Python Bioinformatics
Python bioinformatics combines the power of the Python programming language with the intricacies of biological data analysis. Whether you’re delving into genomics, proteomics, or any other field within bioinformatics, Python offers a versatile toolkit for handling, processing, and analyzing biological data efficiently.
In this article, we’ll explore 20 essential Python codes tailored for beginners in bioinformatics. These examples will cover a range of common tasks encountered in bioinformatics workflows, from sequence manipulation to data visualization.
Also read about Genomic Data Analysis; A Step by Step Guide with Python and R Examples.
1. Reading and Writing Sequence Files
First Python Bioinformatics task is able to open files from external sources like DNA sequence files from FASTA or BLAST. This example demonstrates how to read the contents of a sequence file (such as a FASTA file) using Python’s open() function and then write the contents to a new file. This is useful for accessing and manipulating biological sequence data stored in files.
# Reading a FASTA file
with open('sequence.fasta', 'r') as file:
data = file.read()
# Writing to a new FASTA file
with open('new_sequence.fasta', 'w') as file:
file.write(data)
2. Sequence Length Calculation
Calculating the length of a sequence is a fundamental operation in python bioinformatics. This code snippet utilizes Python’s built-in len() function to determine the length of a DNA, RNA, or protein sequence, aiding in various analyses and computations.
sequence = "ATCGATCGATCG"
length = len(sequence)
print("Sequence Length:", length)
3. Counting Nucleotides
Analyzing the composition of nucleotides (A, T, C, and G) within a DNA sequence is essential in python bioinformatics. By using Python’s string methods, specifically the count() method, one can efficiently count the occurrences of each nucleotide within a sequence.
sequence = "ATCGATCGATCG"
counts = {'A': sequence.count('A'), 'T': sequence.count('T'), 'C': sequence.count('C'), 'G': sequence.count('G')}
print("Nucleotide Counts:", counts)
4. Transcribing DNA to RNA
Transcription is the process of synthesizing an RNA molecule from a DNA template. This code snippet demonstrates how to convert a DNA sequence into an RNA sequence by replacing all occurrences of thymine (T) with uracil (U), mimicking the natural transcription process.
dna_sequence = "ATCGATCGATCG"
rna_sequence = dna_sequence.replace('T', 'U')
print("RNA Sequence:", rna_sequence)
5. Reverse Complement of DNA
The reverse complement of a DNA sequence is often required in various python bioinformatics analyses, such as primer design and sequence alignment. This snippet showcases how to generate the reverse complement by mapping each nucleotide to its complement and then reversing the resulting sequence.
dna_sequence = "ATCGATCGATCG"
reverse_complement = ''.join([{'A': 'T', 'T': 'A', 'C': 'G', 'G': 'C'}[base] for base in reversed(dna_sequence)])
print("Reverse Complement:", reverse_complement)
6. Translating DNA to Protein
Translation is the process of converting a DNA sequence into a corresponding protein sequence, involving the reading of codons and the assignment of amino acids. Using Python’s BioPython library, this code snippet illustrates how to perform this crucial task accurately.
from Bio.Seq import Seq
dna_sequence = "ATCGATCGATCG"
protein_sequence = Seq(dna_sequence).translate()
print("Protein Sequence:", protein_sequence)
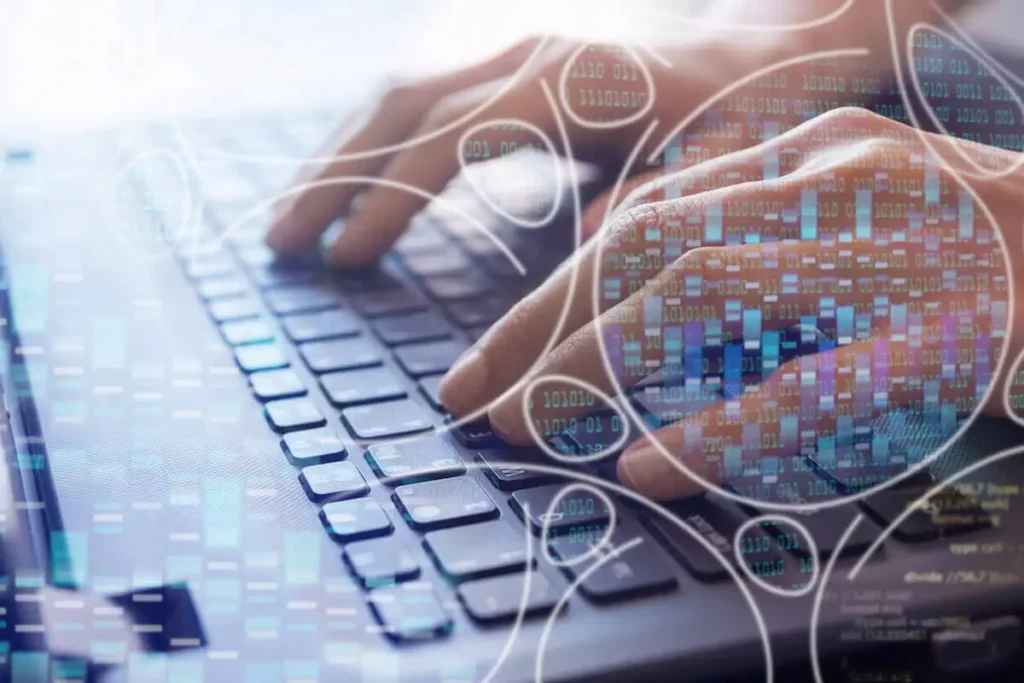
Click here to learn about Top 5 Statistical Programming Languages and Software for Biological Data Science.
7. Calculating GC Content
GC content, the proportion of guanine (G) and cytosine (C) bases in a DNA sequence, is a vital metric in molecular biology. This example demonstrates how to calculate GC content using Python, providing insights into the stability and function of DNA molecules.
sequence = "ATCGATCGATCG"
gc_content = (sequence.count('G') + sequence.count('C')) / len(sequence) * 100
print("GC Content:", gc_content)
8. Reading Sequence Alignments
Sequence alignments are fundamental in python bioinformatics for comparing DNA, RNA, or protein sequences to identify similarities and differences. This code snippet utilizes BioPython’s AlignIO module to read sequence alignment files (e.g., FASTA format) for subsequent analysis.
from Bio import AlignIO
alignment = AlignIO.read("alignment.fasta", "fasta")
print("Alignment Length:", alignment.get_alignment_length())
9. Basic Sequence Alignment
Pairwise sequence alignment is a technique used to align two sequences to identify regions of similarity. This example showcases how to perform a basic sequence alignment using Python’s BioPython library, aiding in evolutionary and functional analyses.
from Bio import pairwise2
seq1 = "ATCGATCG"
seq2 = "ATGGATCG"
alignments = pairwise2.align.globalxx(seq1, seq2)
print("Alignments:", alignments)
10. Parsing GenBank Files
GenBank is a widely used database containing annotated DNA sequences. This code snippet demonstrates how to parse GenBank files using BioPython, enabling researchers to extract valuable biological information such as gene sequences and annotations.
from Bio import SeqIO
record = SeqIO.read("sequence.gb", "genbank")
print("Accession Number:", record.id)
11. Filtering Sequence Data
Filtering sequence data based on specific criteria is common in python bioinformatics workflows. This example illustrates how to filter sequences based on their length using a list comprehension, facilitating data preprocessing and analysis.
sequences = ["ATCGATCG", "ATGGATCG", "ATAGATCG"]
filtered_sequences = [seq for seq in sequences if len(seq) > 8]
print("Filtered Sequences:", filtered_sequences)
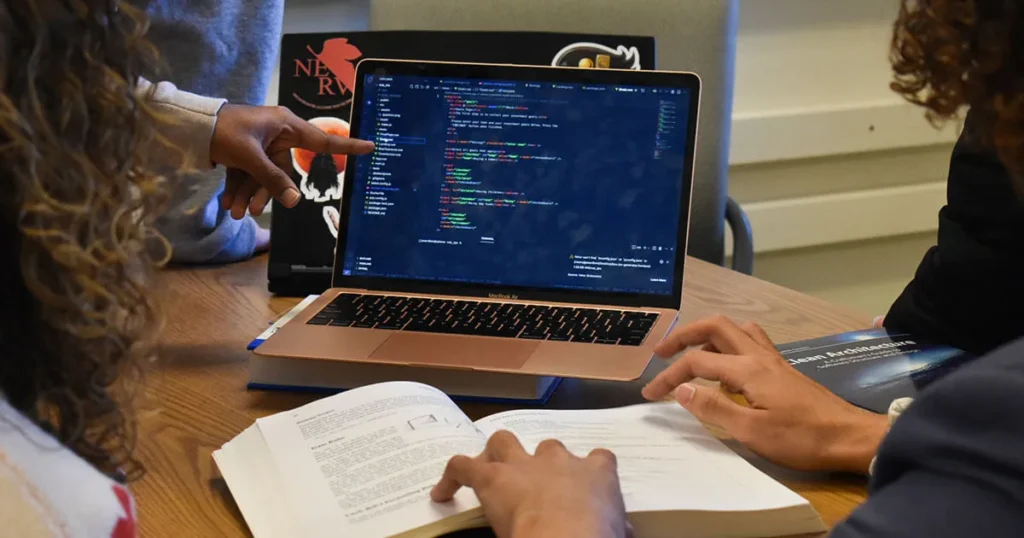
12. Extracting Subsequences
Extracting subsequences from larger sequences is a fundamental task in bioinformatics, useful for focusing analyses on specific regions of interest. Following code showcases how to extract subsequences using Python’s slicing notation.
sequence = "ATCGATCGATCG"
subsequence = sequence[2:6]
print("Subsequence:", subsequence)
13. Handling Multiple Sequence Alignments
Multiple sequence alignments are used to compare and analyze multiple sequences simultaneously, revealing evolutionary relationships and functional conservation. This example demonstrates how to handle multiple sequence alignments using BioPython’s MultipleSeqAlignment class.
from Bio.Align import MultipleSeqAlignment
alignment = MultipleSeqAlignment([])
alignment.add_sequence("seq1", "ATCGATCG")
alignment.add_sequence("seq2", "ATGGATCG")
print("Alignment:", alignment)
14. Parsing PDB Files
Protein Data Bank (PDB) files contain three-dimensional structural information about proteins. This code showcases how to parse PDB files using BioPython’s PDBParser, enabling structural bioinformatics analyses and visualization.
from Bio.PDB import PDBParser
parser = PDBParser()
structure = parser.get_structure("1XYZ", "file.pdb")
print("Structure:", structure)
15. Basic Data Visualization
Data visualization is essential for exploring and communicating biological data effectively. This example utilizes Python’s matplotlib library to create a simple line plot, demonstrating the basics of data visualization in bioinformatics.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [10, 15, 7, 10, 5]
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Sample Plot')
plt.show()
16. Sequence Motif Searching
Sequence motifs are short, recurring patterns in biological sequences that often have functional significance. This snippet illustrates how to search for sequence motifs using regular expressions in python bioinformatics, aiding in motif discovery and analysis.
import re
sequence = "ATCGATCGATCG"
motif = "ATCG"
matches = re.finditer(motif, sequence)
for match in matches:
print("Motif found at:", match.start())
Explore more about 11 Python Packages and Cheat Sheet for Biological Data here.
17. BLAST Sequence Searching
Basic Local Alignment Search Tool (BLAST) is a powerful tool for comparing sequences against a database to identify similar sequences. This code snippet demonstrates how to perform a BLAST search programmatically using Python’s BioPython library.
from Bio.Blast import NCBIWWW
result_handle = NCBIWWW.qblast("blastn", "nt", "ATCGATCGATCG")
print(result_handle.read())
18. Phylogenetic Tree Construction
Phylogenetic trees depict the evolutionary relationships between biological entities, such as species or genes. This example showcases how to construct and visualize phylogenetic trees using BioPython, aiding in evolutionary analysis and classification.
from Bio import Phylo
tree = Phylo.read("tree.nwk", "newick")
Phylo.draw(tree)
19. Statistical Analysis of Biological Data
Statistical analysis is crucial for interpreting biological data and drawing meaningful conclusions. Following code utilizes Python’s numpy library to perform basic statistical calculations such as mean and standard deviation, facilitating quantitative analysis in python bioinformatics.
import numpy as np
data = [1, 2, 3, 4, 5]
mean = np.mean(data)
std_dev = np.std(data)
print("Mean:", mean)
print("Standard Deviation:", std_dev)
20. Machine Learning Applications
Machine learning techniques can be applied to various python bioinformatics tasks, such as sequence classification and prediction. This example illustrates how to use Python’s scikit-learn library to train a support vector machine (SVM) model for predictive modeling in bioinformatics.
from sklearn import svm
from sklearn.model_selection import train_test_split
X = [[0, 0], [1, 1]]
y = [0, 1]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
clf = svm.SVC()
clf
Conclusion
In conclusion, Python bioinformatics offers an extensive array of tools and techniques essential for beginners venturing into the field. Through the examples provided in this article, we’ve explored foundational concepts ranging from sequence manipulation to data visualization and statistical analysis. By leveraging Python’s simplicity and versatility, researchers can efficiently handle, process, and analyze biological data, paving the way for groundbreaking discoveries in genomics, proteomics, and beyond.
As the interdisciplinary field of bioinformatics continues to evolve, mastering these essential Python codes will undoubtedly empower researchers to unravel the complexities of life’s molecular machinery with greater precision and insight.
Read about Bioinformatics vs Biostatistics. A 2024 Analysis of Biological Trends.