If else R programming is a fundamental concept used in controlling the flow of execution based on conditions. This approach allows programmers to execute certain blocks of code only when specified conditions are met.
Understanding how to effectively use if else programming in R is crucial for data analysis, allowing decisions to be automated within scripts.
R is an especially user-friendly language for data science due to its comprehensive statistical packages and built-in functions that streamline complex analyses like t-tests, standard deviations, and partial correlations. The syntax in R is straightforward, allowing for easy implementation of statistical tests with minimal code. Moreover, R’s active community and wealth of tutorials and resources make it accessible for beginners and invaluable for running sophisticated data science tests efficiently.
Basics of If Else R Programming
The syntax for if else R programming starts with the if statement, which tests a condition. If the condition is true, the code within the braces ({}) immediately following the condition is executed. If the condition is false, the code within the else part is executed.
Syntax of If Else in R
if (condition) {
# code to execute if condition is true
} else {
# code to execute if condition is false
}
Step-by-Step Explanation
- Condition: The if statement checks whether the condition is true. This condition can include logical operators like <, >, ==, etc.
- Execution Blocks: If the condition evaluates to true, the first block of code inside the braces after the if statement is executed. Otherwise, the code inside the else braces is executed.
- Running the Code: To execute an if else statement in R, simply write it in the script or command window and run it.
Now, let’s dive deeper into if else R programming through seven examples that range from simple to complex.
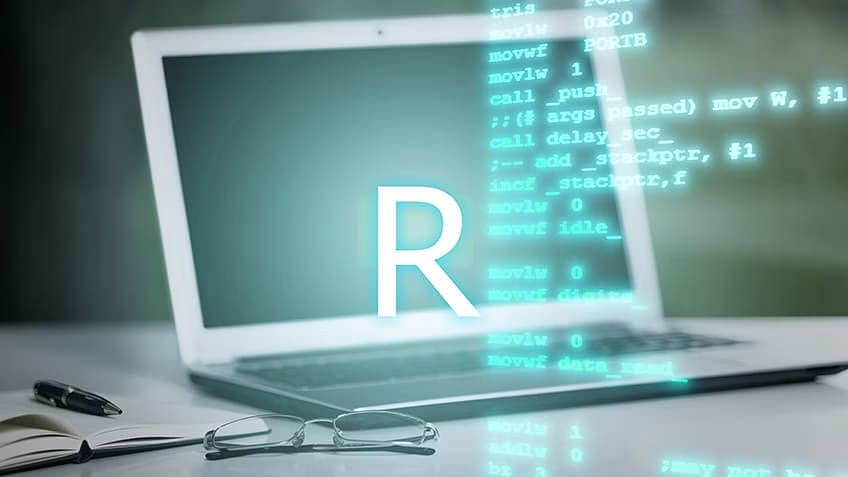
Example 1: Basic If Else R Programming
This simple example checks if a number is positive.
number <- 3
if (number > 0) {
print("The number is positive.")
} else {
print("The number is non-positive.")
}
Output:
[1] "The number is positive."
Example 2: Using Else If
If else programming in R can also handle multiple conditions using else if
number <- 0
if (number > 0) {
print("The number is positive.")
} else if (number < 0) {
print("The number is negative.")
} else {
print("The number is zero.")
}
Output:
[1] "The number is zero."
Example 3: Checking Vector Elements
This example uses if else R programming to check each element of a vector.
numbers <- c(1, -2, 0, 4)
output <- sapply(numbers, function(x) {
if (x > 0) {
"Positive"
} else if (x < 0) {
"Negative"
} else {
"Zero"
}
})
print(output)
Output:
1 -2 0 4
"Positive" "Negative" "Zero" "Positive"
Example 4: Nested If Else
If else R programming can be nested to handle complex logic.
age <- 20
has_permission <- TRUE
if (age >= 18) {
if (has_permission) {
print("Access granted.")
} else {
print("Access denied. Permission required.")
}
} else {
print("Access denied. Age restriction.")
}
Output:
[1] "Access granted."
Example 5: Using If Else with Data Frames
Here, if else R programming checks for specific conditions in a data frame and updates a new column based on these conditions.
library(dplyr)
data <- data.frame(
Name = c("Alice", "Bob", "Charlie"),
Age = c(25, 15, 35)
)
data <- data %>%
mutate(Status = if_else(Age >= 18, "Adult", "Minor"))
print(data)
Output:
Name Age Status
1 Alice 25 Adult
2 Bob 15 Minor
3 Charlie 35 Adult
Example 6: If Else with Loops
This example combines if else R programming with loops to process multiple items in a complex manner.
set.seed(123)
scores <- sample(1:100, 20, replace = TRUE)
results <- lapply(scores, function(score) {
if (score >= 90) {
"Excellent"
} else if (score >= 75) {
"Good"
} else if (score >= 50) {
"Average"
} else {
"Poor"
}
})
names(results) <- scores
print(results)
Output:
... (Output truncated for brevity, will show categorization of scores) ...
Example 7: Complex If Else with Function Definition
The final example of if else programming in R defines a function to assess student grades based on scores and attendance.
evaluate_student <- function(score, attendance) {
if (score >= 85 && attendance >= 90) {
"A"
} else if (score >= 70 && attendance >= 80) {
"B"
} else if (score >= 60 && attendance >= 70) {
"C"
} else {
"F"
}
}
students <- data.frame(
Score = c(88, 75, 66, 45),
Attendance = c(92, 85, 75, 50)
)
students$Grade <- mapply(evaluate_student, students$Score, students$Attendance)
print(students)
Output:
Score Attendance Grade
1 88 92 A
2 75 85 B
3 66 75 C
4 45 50 F
Application of If Else R Programming in Data Science
In data science, if else R programming is a fundamental concept used extensively to make decisions based on data, handle data preprocessing, and derive insights from analysis. It serves multiple roles, from data cleaning to the implementation of complex decision-making algorithms. Here are some of the primary ways if else programming is used in data science:
1. Data Cleaning and Preprocessing
Data often comes with errors, missing values, or outliers. If else R programming can be used to address these issues by implementing conditional checks and transformations. For instance, missing values in a dataset can be replaced with a median or mean, or flagged for further investigation depending on their nature.
Example:
data$Age <- ifelse(is.na(data$Age), median(data$Age, na.rm = TRUE), data$Age)
In this example, missing Age values in a data frame are replaced with the median age, but only where Age is actually missing, showcasing a typical use of if else programming in R for handling missing data.
2. Feature Engineering
Creating new features based on existing data is common in machine learning. If else R programming allows for the creation of categorical variables based on numeric data or the re-categorization of data based on specific criteria.
Example:
data$IncomeLevel <- ifelse(data$Income > 50000, "High", "Low")
This use of if else R programming segments individuals into “High” and “Low” income levels based on their income, aiding in the development of models that might predict behavior based on income.
3. Logical Flow in Analysis
Often, data analysis requires conditional logic to differentiate the analytical approach based on attributes of the data. If else programming enables branching paths where different types of analysis are performed based on certain criteria.
Example:
if (variance(data$Sales) > threshold) {
analysis <- lm(Sales ~ ., data = data)
} else {
analysis <- lm(log(Sales) ~ ., data = data)
}
This approach uses if else R programming to choose between a linear model and a logarithmic transformation of the target variable depending on the variance, which might be important for model accuracy.
4. Handling Exceptions and Errors
In data processing scripts, errors need to be managed to prevent crashes or incorrect outputs. If else programming can ensure that the script handles different types of data inputs gracefully, applying different rules or warnings.
Example:
if (class(data$date) != "Date") {
stop("Data error: date column not in Date format")
}
In this context, if else programming in R is used to check data types before proceeding with date-related operations, preventing runtime errors.
5. Automating Model Selection and Tuning
When building predictive models, it’s often necessary to compare several models or configurations. If else programming facilitates this by allowing conditional execution of different modeling strategies based on performance metrics.
Example:
model1 <- lm(Sales ~ ., data = training_set)
model2 <- lm(log(Sales) ~ ., data = training_set)
if (summary(model1)$r.squared > summary(model2)$r.squared) {
best_model <- model1
} else {
best_model <- model2
}
This example demonstrates the use of if else R programming to select the best performing model based on the R-squared value.
6. Dynamic Reporting
In generating reports or visualizations, you might want different content based on the data. If else statements can change what’s included in a report or how data is presented, based on predefined conditions.
Example:
if (mean(data$Sales) > target) {
print("Target achieved")
} else {
print("Target not achieved")
}
In reporting scenarios, if else programming in R can provide conditional messages based on performance against targets, adding dynamic elements to static reports.
Conclusion
If else programming in R is a versatile tool that can be applied in various data analysis scenarios. From simple condition checks to complex decision-making processes involving data frames and function definitions, mastering if else R programming enhances the ability to perform nuanced data operations efficiently.