Genomics is the study of genomes, the complete set of DNA within an organism. Understanding genomes can lead to breakthroughs in medicine, agriculture, and biology. Python, a versatile and powerful programming language, has become a popular tool in genomics. Its simplicity and extensive libraries make it ideal for handling complex biological data. This article explores the utilization of python for genomics, highlighting key libraries and providing examples.
Why Use Python for Genomics?
Python usage for genomics is popular for several reasons:
Ease of Use
Python programming for genomics is favored because its syntax is clear and easy to learn. This is crucial for biologists who may not have extensive programming backgrounds.
Extensive Libraries
Python coding for genomics boasts a wide range of libraries specifically designed for scientific computing and data analysis. These libraries simplify the process of working with genomic data.
Community Support
A strong community of bioinformaticians and developers supports python for genomics. This community continuously develops new tools, packages and libraries in python for bioinformatics.
Key Python Libraries for Genomics
Several python libraries are essential for genomics work. Here are some of the most widely used:
Biopython
Biopython is a collection of tools for biological computation. It provides functionalities for reading and writing different sequence file formats, performing sequence analysis, and working with biological databases. It is a cornerstone for bioinformatics beginners in python.
Example
from Bio import SeqIO
# Reading a FASTA file
for record in SeqIO.parse("example.fasta", "fasta"):
print(record.id)
print(record.seq)
Pandas
Pandas is a powerful data manipulation library. It is especially useful for handling large genomic datasets stored in tabular formats, such as CSV files. Pandas plays a crucial role in python for genomics research
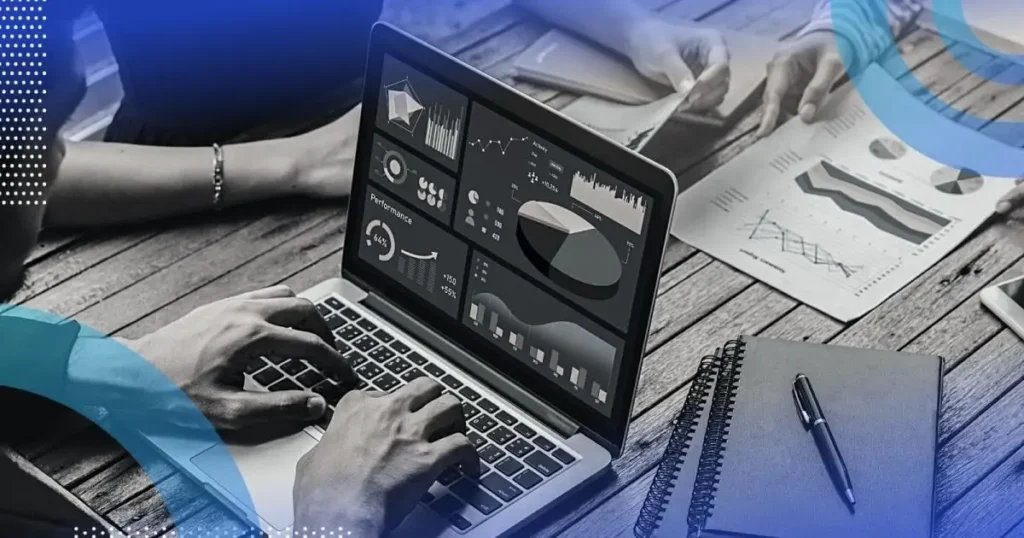
Example
import pandas as pd
# Reading a CSV file
df = pd.read_csv("genomic_data.csv")
print(df.head())
NumPy
NumPy is a library for numerical computing. It provides support for large arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is indispensable in python for genomics data.
Example
import numpy as np
# Creating a NumPy array
data = np.array([1, 2, 3, 4, 5])
print(data)
SciPy
SciPy builds on NumPy and provides additional tools for scientific computing. It includes modules for statistics, optimization, and more, making it essential for genomics data analysis.
Example
from scipy import stats
# Performing a t-test
t_stat, p_val = stats.ttest_1samp(data, 3)
print(f"T-statistic: {t_stat}, P-value: {p_val}")
Matplotlib and Seaborn
Matplotlib and Seaborn are libraries for data visualization. They allow for the creation of complex plots and graphs, which are essential for interpreting genomic data. These libraries are integral to python for genomics data.
Example
import matplotlib.pyplot as plt
import seaborn as sns
# Creating a simple plot
plt.plot(data)
plt.show()
# Creating a more complex plot with Seaborn
sns.histplot(data)
plt.show()
scikit-learn
scikit-learn is a machine learning library. It includes simple and efficient tools for data mining and data analysis, making it ideal for building predictive models with genomic data. scikit-learn is a key component of python for genomics.
Example
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Splitting data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Training a Random Forest model
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
print(predictions)
Applications of Python for Genomics
Python is used in various applications, from sequence analysis to data visualization. Here are some key applications:
Sequence Analysis
Sequence analysis is fundamental in genomics. It involves identifying, analyzing, and comparing DNA, RNA, or protein sequences. Python usage for genomics simplifies these tasks through libraries like Biopython.
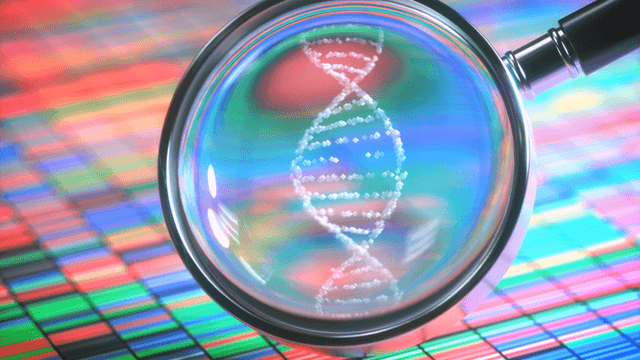
Example: Sequence Alignment
from Bio import pairwise2
# Aligning two sequences
alignments = pairwise2.align.globalxx("ACGT", "ACCT")
for alignment in alignments:
print(pairwise2.format_alignment(*alignment))
Sequence alignment is the process of arranging sequences to identify regions of similarity. This can provide insights into functional, structural, or evolutionary relationships. Python for genomics makes sequence alignment straightforward.
Genome Assembly
Genome assembly is the process of reconstructing the original genome from short DNA sequences. Python for genomics libraries like Biopython can be used to handle and manipulate these sequences.
Example: Assembling Reads
from Bio.Sequencing import Ace
# Reading an ACE file
with open("assembly.ace") as handle:
for contig in Ace.parse(handle):
print(contig.name)
for read in contig.reads:
print(read.rd.name)
Variant Calling
Variant calling identifies variants from sequence data. These variants can be linked to diseases or traits. Python for genomics libraries like pysam can be used to manipulate and analyze sequence alignment/map (SAM) files.
Example: Reading a BAM File
import pysam
# Opening a BAM file
samfile = pysam.AlignmentFile("example.bam", "rb")
for read in samfile.fetch():
print(read)
Data Visualization
Visualizing genomic data helps in understanding and interpreting complex datasets. Python for genomics libraries like Matplotlib and Seaborn are commonly used for this purpose.
Example: Visualizing Variant Frequencies
import matplotlib.pyplot as plt
# Variant frequencies
variants = {"A": 50, "T": 30, "C": 10, "G": 10}
# Creating a bar chart
plt.bar(variants.keys(), variants.values())
plt.xlabel("Variants")
plt.ylabel("Frequency")
plt.title("Variant Frequencies")
plt.show()
Machine Learning in Genomics
Machine learning models can predict outcomes based on genomic data. Python for genomics uses scikit-learn to build and evaluate these models.
Example: Predicting Disease Susceptibility
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Example dataset
X = [[0.1, 0.2], [0.2, 0.1], [0.3, 0.4], [0.4, 0.3]]
y = [0, 0, 1, 1]
# Splitting data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.5)
# Training a Random Forest model
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
print(predictions)
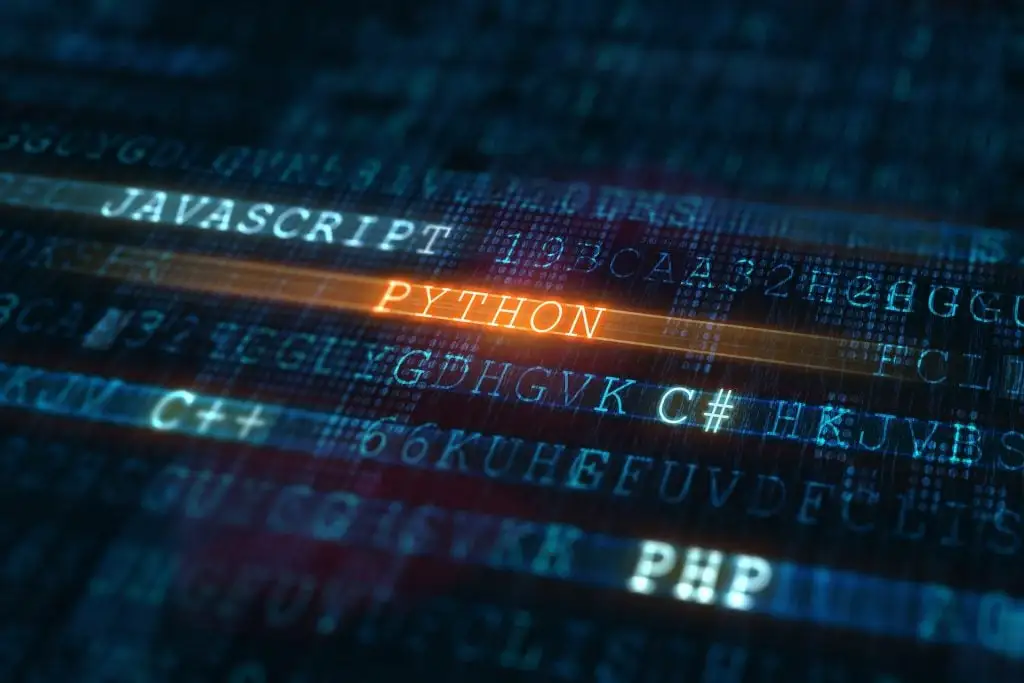
Challenges and Future Directions of Python for Genomics
While python is powerful, there are challenges in its use. The primary challenges include handling large datasets, integrating with other tools, and ensuring reproducibility.
Handling Large Datasets
Genomic data can be enormous. Efficiently handling and analyzing these datasets requires optimized code and sometimes the use of high-performance computing resources. Python for genomics can leverage libraries like Dask for better performance.
Example: Using Dask for Large Datasets
import dask.dataframe as dd
# Reading a large CSV file
df = dd.read_csv("large_genomic_data.csv")
print(df.head())
Dask is a library for parallel computing in python, which can handle large datasets more efficiently, making it valuable for genomics.
Integration with Other Tools
Genomics often involves using multiple tools and languages. Integrating python with other tools can be complex but necessary for comprehensive analyses.
Example: Calling R from Python
import rpy2.robjects as ro
# Calling an R function
ro.r('x <- rnorm(10)')
x = ro.r('x')
print(x)
rpy2 is a python library that allows for calling R functions from python, enhancing the versatility of python for genomics.
Ensuring Reproducibility
Reproducibility is crucial in scientific research. Documenting code and using version control systems like Git can help ensure that analyses are reproducible. Python for genomics can be made more reproducible using tools like Jupyter Notebooks.
Example: Using Jupyter Notebooks
# Starting a Jupyter Notebook
!jupyter notebook
Jupyter Notebooks allow for writing and documenting code in an interactive environment, which is beneficial for genomics analysis in python.
Conclusion
Python for genomics has become a cornerstone in the field of genomics due to its simplicity, extensive libraries, and strong community support. It facilitates various genomic applications, from sequence analysis to data visualization and machine learning. Despite challenges like handling large datasets and ensuring reproducibility, python continues to be an invaluable tool for genomic research.